JSON Web Tokens (JWTs) embed claims and metadata in a signed structure for authentication and authorization. After verifying user credentials, the server issues a JWT that the client sends in subsequent requests, typically as a Bearer token in the Authorization header. This method eliminates the need to resend credentials with every request.
When included in a HTTP header, the server validates the JWT’s signature and claims. If valid, it grants access to protected resources without external lookups, reducing overhead and simplifying distributed architectures.
Integrating JWTs with cURL involves retrieving a token, attaching it to each request, and securely storing and refreshing it as needed. Proper JWT handling supports scalable, secure, and high-performance web systems.
Steps to authenticate using JWT in cURL:
- Open a terminal.
- Obtain the JWT by sending credentials to the authentication endpoint.
$ curl --data '{"username":"user","password":"pass"}' --header "Content-Type: application/json" "http://example.com/auth/login" {"token":"eyJhbGciOi..."}
The server returns a JWT if credentials are valid. Use the actual token from the response.
- Include the JWT in the Authorization header as a Bearer token.
$ curl --header "Authorization: Bearer eyJhbGciOi..." "http://example.com/api/protected" { "data": "protected content" }
Replace the ellipsis with the full JWT.
- Ensure the Bearer schema is used as expected by the server.
Bearer is the correct prefix when using JWT-based headers.
- Verify correct inclusion of the Authorization header.
$ curl --header "Authorization: Bearer <your_jwt_here>" "http://example.com/api/protected" --verbose > Authorization: Bearer <your_jwt_here> < HTTP/1.1 200 OK
--verbose confirms the header’s presence and validity.
- Handle errors like 401 or 403 by checking token validity and expiration.
Refresh the JWT if necessary.
- Refresh the JWT using the refresh token endpoint.
$ curl --data '{"refreshToken":"<your_refresh_token>"}' --header "Content-Type: application/json" "http://example.com/auth/refresh" {"token":"new_jwt_here"}
A successful refresh returns a new JWT.
- Automate requests with scripts for continuous secure access.
Use tooling to streamline authentication and token refreshes.
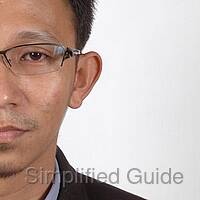
Mohd Shakir Zakaria is a cloud architect with deep roots in software development and open-source advocacy. Certified in AWS, Red Hat, VMware, ITIL, and Linux, he specializes in designing and managing robust cloud and on-premises infrastructures.
Comment anonymously. Login not required.