Bearer tokens are issued after successful authentication, often via OAuth 2.0. Instead of sending a username and password each time, the client includes a short-lived Bearer token in the Authorization header, enhancing both security and flexibility.
The Bearer token grants repeated secure access until it expires. With cURL, placing the token in the Authorization header confirms the request is authenticated. Because tokens expire, a refresh process ensures continuous access.
Protecting tokens is essential. Storing the Bearer token in environment variables or files and refreshing it regularly prevents unauthorized access and maintains smooth interaction with token-based APIs.
Steps to authenticate with bearer token in cURL:
- Open a terminal.
- Acquire the Bearer token from the authentication provider.
$ curl --data "client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&grant_type=client_credentials" "https://auth.example.com/oauth/token" { "access_token": "YOUR_ACCESS_TOKEN", "token_type": "Bearer", "expires_in": 3600 }
Replace YOUR_CLIENT_ID and YOUR_CLIENT_SECRET with valid credentials.
- Include the Bearer token in the Authorization header for requests.
$ curl --header "Authorization: Bearer YOUR_ACCESS_TOKEN" "https://api.example.com/data" { "data": "sample data response" }
Prefix the token with Bearer followed by a space.
- Use --verbose to verify the token’s presence.
$ curl --header "Authorization: Bearer YOUR_ACCESS_TOKEN" "https://api.example.com/data" --verbose > Authorization: Bearer YOUR_ACCESS_TOKEN
--verbose displays request headers and responses for debugging.
- Store the Bearer token in a secure file.
$ echo "Authorization: Bearer YOUR_ACCESS_TOKEN" > .bearer_token
Protect this file to prevent token theft.
- Refresh the Bearer token when it expires.
$ curl --data "client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN" "https://auth.example.com/oauth/token" { "access_token": "NEW_ACCESS_TOKEN", "token_type": "Bearer", "expires_in": 3600 }
Use the refresh token endpoint to obtain a new Bearer token.
- Load the authorization header from the file as needed.
$ curl --config .bearer_token "https://api.example.com/data" { "data": "sample data response" }
--config simplifies repeated requests using stored headers.
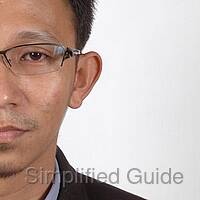
Mohd Shakir Zakaria is a cloud architect with deep roots in software development and open-source advocacy. Certified in AWS, Red Hat, VMware, ITIL, and Linux, he specializes in designing and managing robust cloud and on-premises infrastructures.
Comment anonymously. Login not required.