Resolving a hostname from an IP address is a common task in network management and development. It involves performing a reverse DNS lookup to map an IP address to its corresponding hostname. This process is essential for identifying machines within a network.
In Python, the socket module provides the functionality to accomplish this task using the gethostbyaddr function. This function takes an IP address and returns the associated hostname, also known as the Fully Qualified Domain Name (FQDN). This is useful in various scenarios, such as network logging, security analysis, and troubleshooting.
This guide will show you how to use Python to retrieve hostnames from IP addresses. The steps include using the socket module and writing a simple script to automate the process. This approach is efficient and straightforward for technical users.
Steps to get domain name from IP address in Python:
- Launch your preferred Python shell.
$ ipython3 Python 3.8.2 (default, Apr 27 2020, 15:53:34) Type 'copyright', 'credits' or 'license' for more information IPython 7.13.0 -- An enhanced Interactive Python. Type '?' for help.
- Import socket module.
In [1]: import socket
- Use socket.gethostbyaddr to get host name from an IP address.
In [2]: socket.gethostbyaddr('93.184.216.34') Out[2]: ('www.example.com', [], ['93.184.216.34'])
Hostname in this context refers to Fully Qualified Domain Name or FQDN.
- Create a Python script that accepts an IP address as parameter and outputs corresponding host information.
- ip-to-hostname.py
#!/usr/bin/env python3 import socket import sys address = sys.argv[1] host = socket.gethostbyaddr(address) print('Address: ', address, '\n' 'Host: ', host)
- Run the script from the command line and provide an IP address as a parameter.
$ python3 ip-to-hostname.py 216.58.196.4 Address: 216.58.196.4 Host: ('kul08s09-in-f4.1e100.net', [], ['216.58.196.4'])
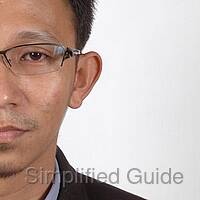
Mohd Shakir Zakaria is a cloud architect with deep roots in software development and open-source advocacy. Certified in AWS, Red Hat, VMware, ITIL, and Linux, he specializes in designing and managing robust cloud and on-premises infrastructures.
Comment anonymously. Login not required.