Python supports converting hostname or domain name to IP addresses without using an external program. The feature is provided by the gethostbyname function from the socket module.
gethostbyname outputs a single IP address for a given domain. If the host or domain has multiple IP addresses, you can use gethostbyname_ex(). gethostbyname_ex() returns the IP list as an array.
You can use gethostbyname and gethostbyname_ex() to resolve a hostname to IP address in Python by using the interactive shell or within a script.
Steps to get IP address from hostname or domain in Python:
- Launch your favourite Python shell.
$ ipython3 Python 3.8.2 (default, Apr 27 2020, 15:53:34) Type 'copyright', 'credits' or 'license' for more information IPython 7.13.0 -- An enhanced Interactive Python. Type '?' for help.
- Import socket module.
In [1]: import socket
- Get IP from hostname by calling socket.gethostbyname function with hostname as parameter .
In [2]: socket.gethostbyname('www.google.com') Out[2]: '216.58.196.4'
Hostname in this context refers to Fully Qualified Domain Name or FQDN.
Use gethostbyname_ex() instead if the domain has multiple IP addresses.
- Create a Python script that accepts hostname as parameter and outputs IP address such as the following.
- hostname-to-ip.py
#!/usr/bin/env python3 import socket import sys hostname = sys.argv[1] ip = socket.gethostbyname(hostname) print('Hostname: ', hostname, '\n' 'IP: ', ip)
- Run the script from the command line with hostname as a parameter to test.
$ python3 hostname-to-ip.py 'www.google.com' Hostname: www.google.com IP: 172.217.31.68
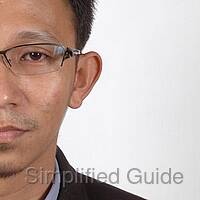
Author: Mohd
Shakir Zakaria
Mohd Shakir Zakaria is an experienced cloud architect with a strong development and open-source advocacy background. He boasts multiple certifications in AWS, Red Hat, VMware, ITIL, and Linux, underscoring his expertise in cloud architecture and system administration.

Mohd Shakir Zakaria is an experienced cloud architect with a strong development and open-source advocacy background. He boasts multiple certifications in AWS, Red Hat, VMware, ITIL, and Linux, underscoring his expertise in cloud architecture and system administration.
Discuss the article:
Comment anonymously. Login not required.