Extracting the host name from a URL in PHP is a common task in web development. The host name is the domain part of a URL, such as example.com in https://www.example.com/page. By using built-in PHP functions, you can easily obtain the host name from any given URL. This is useful for tasks like URL validation, parsing, and handling different domain-specific logic.
To extract the host name from a URL, you can use the parse_url() function. This function breaks down a URL into its components, including the scheme, host, path, and more. By accessing the host component, you can retrieve the domain name.
Steps to get host name from URL:
- Use the parse_url() function to parse the URL.
$url = "https://www.example.com/page"; $parsedUrl = parse_url($url);
- Access the host component from the result of parse_url().
$hostName = $parsedUrl['host'];
- Store the host name in a variable for further use.
echo "Host name: " . $hostName;
- Implement error handling to manage invalid URLs.
if (isset($parsedUrl['host'])) { echo "Host name: " . $hostName; } else { echo "Invalid URL or host component not found."; }
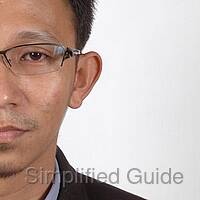
Author: Mohd
Shakir Zakaria
Mohd Shakir Zakaria is a cloud architect with deep roots in software development and open-source advocacy. Certified in AWS, Red Hat, VMware, ITIL, and Linux, he specializes in designing and managing robust cloud and on-premises infrastructures.

Mohd Shakir Zakaria is a cloud architect with deep roots in software development and open-source advocacy. Certified in AWS, Red Hat, VMware, ITIL, and Linux, he specializes in designing and managing robust cloud and on-premises infrastructures.
Discuss the article:
Comment anonymously. Login not required.