Optimizing query performance in MySQL and MariaDB is crucial for maintaining efficient and responsive database-driven applications. As the amount of data grows, it becomes increasingly important to ensure that queries are executed quickly and with minimal resource consumption. This guide will provide a step-by-step process to optimize query performance in MySQL or MariaDB by analyzing and improving query design, indexing, and other database configuration settings.
To achieve optimal performance, it is essential to identify and address potential bottlenecks in the query execution process. By examining the query plan, understanding the data distribution, and adjusting the database configuration, you can significantly improve the performance of your queries.
This guide assumes you have a basic understanding of SQL and database concepts. Follow the steps outlined below to improve the performance of your MySQL or MariaDB queries, ensuring your applications remain fast and responsive even as your data scales.
Steps to optimize MySQL or MariaDB query performance:
- Identify slow queries using the slow query log by enabling it in your MySQL or MariaDB configuration file.
[mysqld] slow_query_log = 1 slow_query_log_file = /var/log/mysql-slow.log long_query_time = 1
- Analyze the slow query log using tools like mysqldumpslow or pt-query-digest to find problematic queries.
- Use the EXPLAIN statement to analyze the query execution plan, revealing potential performance issues.
EXPLAIN SELECT * FROM users WHERE last_name = 'Smith';
- Optimize your queries by reducing the number of rows scanned, using more selective conditions, and avoiding functions in the WHERE clause.
- Create indexes on frequently searched columns to speed up query execution.
CREATE INDEX idx_last_name ON users (last_name);
- Remove unused or redundant indexes to reduce the overhead of index maintenance and updates.
- Optimize JOIN operations by using appropriate join types, such as INNER JOIN or OUTER JOIN, and leveraging indexes on joined columns.
- Use pagination or limit the number of rows returned in a result set to reduce the amount of data processed.
SELECT * FROM users LIMIT 10 OFFSET 20;
- Optimize the database schema by normalizing tables and splitting large tables into smaller ones using partitioning.
- Update your MySQL or MariaDB configuration to allocate more memory to buffers and caches, such as the innodb_buffer_pool_size and query_cache_size settings.
- Regularly monitor and optimize your tables using the ANALYZE TABLE, OPTIMIZE TABLE, and CHECK TABLE commands.
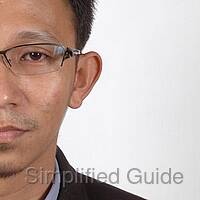
Mohd Shakir Zakaria is an experienced cloud architect with a strong development and open-source advocacy background. He boasts multiple certifications in AWS, Red Hat, VMware, ITIL, and Linux, underscoring his expertise in cloud architecture and system administration.
Comment anonymously. Login not required.